All examples have been derived from
See the comments in the code for explanation of the use of the different input modes. I hope that the code is understandable. The example for canonical input is commented best, the other examples are commented only where they differ from the example for canonical input to emphasize the differences.
Perform a read on the serial port. Reading input from serial port is done by Listen invoked right after initialization of the serial port. We do this in the sample code by creating an async read task using the DataReader object that waits on the InputStream of the SerialDevice object. Due to differences in handling concurrent tasks, the. 12F675 Tutorial 3: PIC Serial Port. Note: It is not worth trying to code the value into the C source as it will use a non-standard method of programming. Alternatively download winpic800 (look online to download it) and set the device to 12F675, load up the hex file and go to the memory location in winpic800 and type in the last location.
The descriptions are not complete, but you are encouraged to experiment with the examples to derive the best solution for your application.
Don't forget to give the appropriate serial ports the right permissions (e. g.:
- In today’s programming tutorial, I am going to describe some basics about how we can perform serial port communication from our C#.NET applications. Serial communications can be done via either direct to physical serial port connected to the computer or via a USB to serial converter interface. If the device do require a serial port and your.
- I was using the Dev C compiler to write the code. Now I am trying to verify the output.What I did was to write the letter 'a' to the serial port. The serial port is connected to a digital oscilloscope and I intend to see the voltage patterns that correspond to the letter 'a' as below.
In non-canonical input processing mode, input is not assembled into lines and input processing (erase, kill, delete, etc.) does not occur. Two parameters control the behavior of this mode:
If MIN > 0 and TIME = 0, MIN sets the number of characters to receive before the read is satisfied. As TIME is zero, the timer is not used.
If MIN = 0 and TIME > 0, TIME serves as a timeout value. The read will be satisfied if a single character is read, or TIME is exceeded (t = TIME *0.1 s). If TIME is exceeded, no character will be returned.

If MIN > 0 and TIME > 0, TIME serves as an inter-character timer. The read will be satisfied if MIN characters are received, or the time between two characters exceeds TIME. The timer is restarted every time a character is received and only becomes active after the first character has been received.
If MIN = 0 and TIME = 0, read will be satisfied immediately. The number of characters currently available, or the number of characters requested will be returned. According to Antonino (see contributions), you could issue a
Serial Port Communications Software

By modifying
Serial Port Communication Program
3.3. Asynchronous Input3.4. Waiting for Input from Multiple SourcesThis section is kept to a minimum. It is just intended to be a hint, and therefore the example code is kept short. This will not only work with serial ports, but with any set of file descriptors.
The select call and accompanying macros use a
The given example blocks indefinitely, until input from one of the sources becomes available. If you need to timeout on input, just replace the select call by:
This example will timeout after 1 second. If a timeout occurs, select will return 0, but beware that
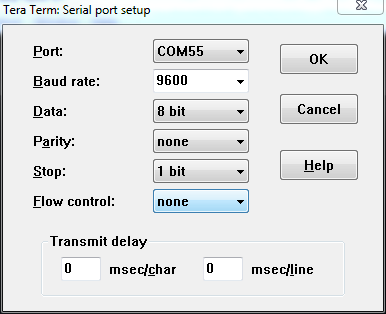
C Source Code Serial Port Communication Tutorial Free
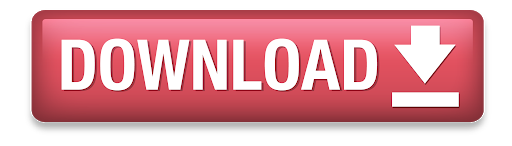